
User: Vaibhav Rananaware – March 1, 2023
One of the fundamental concepts in LWC development is understanding lifecycle hooks – methods that allow developers to tap into various stages of a component’s lifecycle. In this blog post, we’ll delve into the intricacies of LWC lifecycle hooks, exploring their significance, functionality, and practical applications within the Salesforce ecosystem.
Also Read: QR Code Generation using Formula field or LWC Component in Salesforce
What are Lifecycle Hooks?
Lifecycle hooks in LWC provide developers with the ability to execute custom logic at specific moments during the lifecycle of a component. These hooks enable fine-grained control over component initialization, rendering, updates, and destruction. By leveraging lifecycle hooks, developers can enhance the functionality, performance, and user experience of their Salesforce applications.
The lifecycle of a Lightning Web Component unfolds in distinct stages. At each stage, we can do something special to change how the component works. Let’s explore these stages more closely to understand how we can make our components behave just the way we want.
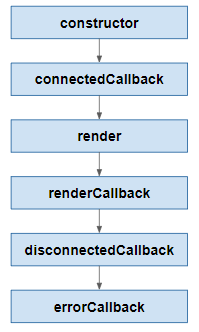
Stages of Life Cycle Hooks in LWC
Creation and Initialization
constructor(): The journey begins with the constructor hook, where the component is initialized. The constructor is called when a component is created but before it’s connected to the DOM. This stage is perfect for setting up initial properties and establishing a baseline state for the component.
This hook flows from parent to child, which means that it fires in the parent first. You can’t access child
elements because they don’t exist yet. Properties aren’t passed yet, either. Properties are assigned to the component after construction and before the connectedCallback() hook.
import { LightningElement } from ‘lwc’; export default class ConstructorExample extends LightningElement { constructor() { super(); console.log(‘Constructor called’); } } |
Component Insertion
connectedCallback(): As the component finds its place in the DOM, the connectedCallback hook gets invoked. This stage offers a prime opportunity to execute one-time setup tasks, such as fetching initial data or configuring event listeners.
This hook flows from parent to child. We can’t access child elements because they don’t exist yet. The connectedCallback() hook can fire more than one time. If we remove an element and then insert it into another position, such as when you reorder a list, the hook fires several times.
import { LightningElement } from ‘lwc’; export default class ConnectedCallbackExample extends LightningElement { connectedCallback() { console.log(‘Connected callback called’); } } |
Rendering and Updates
render(): This method is not technically a lifecycle hook. It is a protected method on the LightningElement class, it may be called before or after connectedCallback(). It’s rare to call render() in a component. The main use case is to conditionally render a template. Define business logic to decide which template (HTML file) to use. The method must return a valid HTML template.
renderedCallback(): Following the rendering phase, the renderedCallback hook provides a post-rendering sanctuary. It is called after every render of the component, which means both after the first render and after any subsequent rerenders. You can use it to perform post-rendering logic or to manipulate the DOM directly. It enables interaction with the rendered DOM, facilitating dynamic updates or additional rendering tasks. If we use renderedCallback() to perform a one-time operation, you must track it manually (using an initialRender private property, for example). If you perform changes to reactive attributes, guard them or they can trigger wasteful rerenders or an infinite rendering loop.
import { LightningElement } from ‘lwc’; export default class RenderedCallbackExample extends LightningElement { renderedCallback() { console.log(‘Rendered callback called’); } } |
Component Destruction
disconnectedCallback(): The disconnectedCallback invokes when the element is removed from a document. This hook flows from parent to child. disconnectedCallback() is used to clean up work done in the connectedCallback(), like purging caches or removing event listeners. We can also use this hook to unsubscribe from a message channel. Here, developers bid farewell to the component, performing cleanup tasks and releasing resources before its removal from the DOM.
import { LightningElement } from ‘lwc’; export default class DisconnectedCallbackExample extends LightningElement { disconnectedCallback() { console.log(‘Disconnected callback called’); } } |
Error Handling
errorCallback(error, stack): In the event of errors during rendering, lifecycle methods, or event handlers, the errorCallback swoops in to save the day. This stage empowers developers to handle errors gracefully, ensuring a seamless user experience.
The error boundary component’s errorCallback() lifecycle hook to log stack information and render an alternative view to tell users what happened and what to do next. The method works like a JavaScript catch{} block for components that throw errors in their lifecycle hooks or in their event handlers declared in an HTML template.
import { LightningElement } from ‘lwc’; export default class ErrorCallbackExample extends LightningElement { errorCallback(error, stack) { console.error(‘Error callback called with error:’, error); } } |
Also Read: Discover Salesforce Spring ’24 Exciting New Features